How to add SurveyJS React to Gatsby
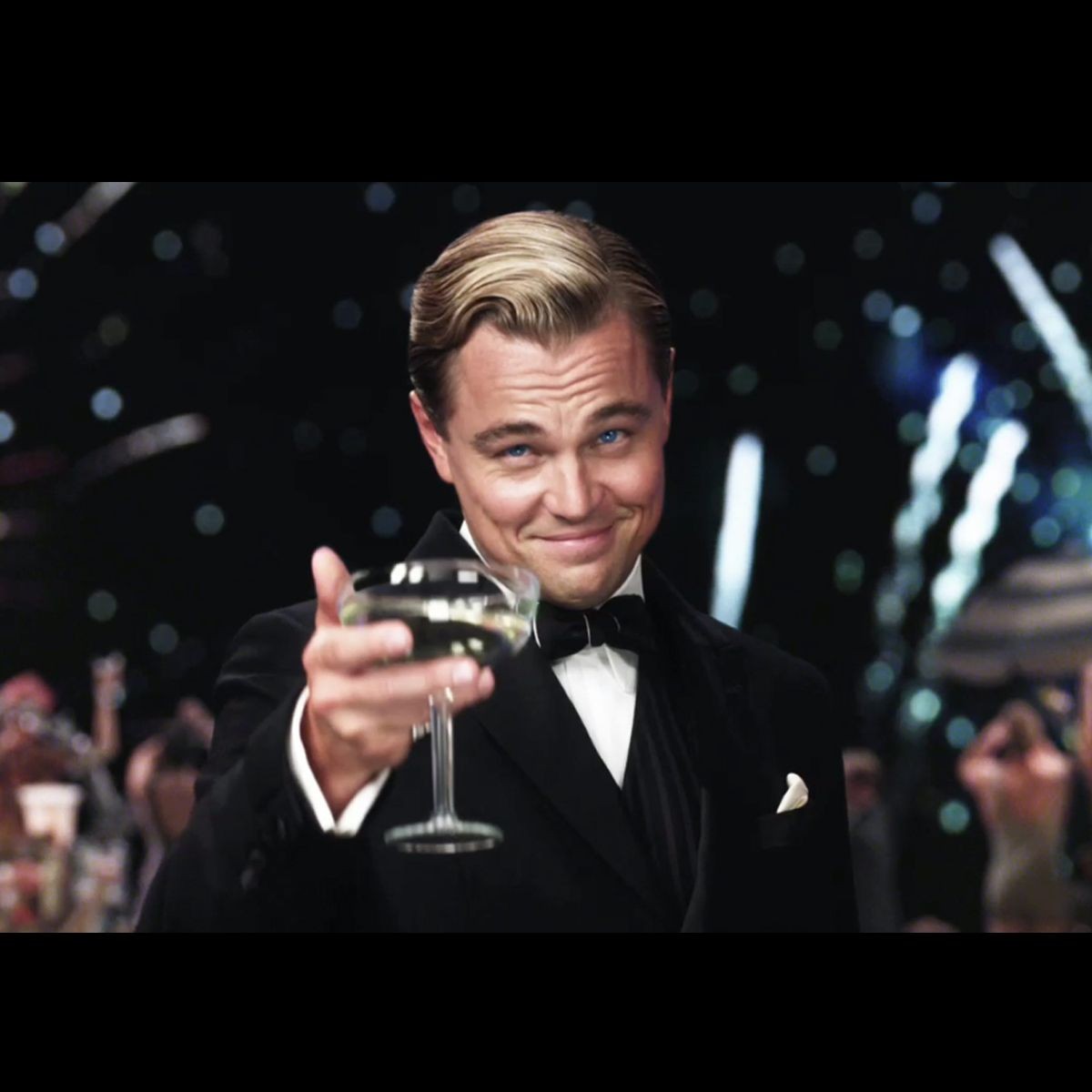
-
I assume you already created a Gatsby app by following the Quick Start documentation
-
Create your survey here.
-
Click on ‘Embed Survey’ and copy only the surveyJSON. Example:
const surveyJSON = { pages: [ { name: "page1", elements: [ { type: "text", name: "question1", title: "Firstname", isRequired: true, }, ], title: "Survey", description: "Please give me your data.", }, ], };
-
Install survey-react in your Gatsby project.
npm install survey-react
-
Create a component Form.js in pages > components with the following content:
import * as React from "react"; import * as Survey from "survey-react"; import "survey-react/survey.css"; export const Form = () => { // Paste your surveyJSON here const surveyJSON = { ... } //Define a callback methods on survey complete const onComplete = (survey) => { //Write survey results into database console.log("Survey results: " + JSON.stringify(survey.data)); }; return <Survey.Survey json={json} onComplete={onComplete} />; };
-
Call the component in your index.js Start the local development server with
npm run develop